Assignment 4: Watch where you point!
Due Friday, February 14th, before midnight
The goals for this assignment are:
-
Use malloc and free
-
Work with pointer-based data structures
-
Use gdb and valgrind.
All programs must run without memory errors and leaks!
1. Update your repository
Do a fetch upstream to obtain the basecode for this assignment.
Using the command line
-
Open terminal and change your current directory to your assignment repository.
-
Run the command
git fetch upstream
-
Run the command
git merge upstream/main
Your repository should now contain a new folder named A04
.
The fetch
and merge
commands update your repository with any changes from the original.
2. Magic Square
Implement a program, magic_square.c
, that tests whether a given matrix is a magic square.
A magic square is an N by N matrix where each
row, column, and diagonal has the same sum, called the magic constant.
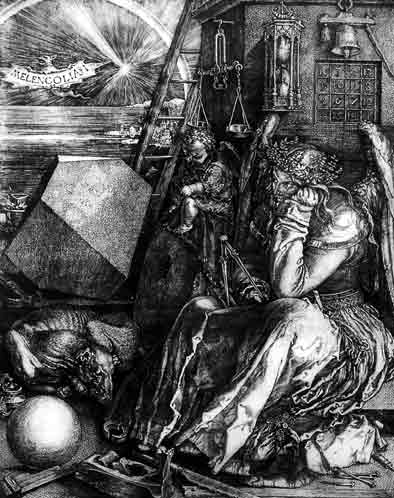
$ make magic_square
gcc -g magic_square.c -o magic_square
$ ./magic_square < magic1.txt
8 1 6
3 5 7
4 9 2
M is a magic square (magic constant = 15)
$ ./magic_square < magic2.txt
9 1 6
3 5 7
4 8 2
M is NOT a magic square!
$ ./magic_square < magic3.txt
1
M is a magic square (magic constant = 1)
Requirements/Hints:
-
Read in the matrix from stdin using
scanf(" %d", &val);
. The first two values are the dimensions of the matrix. All subsequent integers are the values of the matrix. -
Use malloc and free to allocate a 2D array to store the inputs.
3. Sorted Snacks
Implement a program, sorted_snackbar.c
, that allows users to add snacks to the snackbar and displays
them in alphabetical order. Your program should have the same features as last week’s snackbar, but should
use a linked list to store the snacks, rather than an array.
$ make sorted_snackbar
gcc sorted_snackbar.c -o sorted_snackbar
$ ./sorted_snackbar
Enter a number of snacks: 3
Enter a name: Slurm
Enter a cost: 1.50
Enter a quantity: 3
Enter a name: Beans
Enter a cost: 5
Enter a quantity: 1
Enter a name: Carrots
Enter a cost: 2
Enter a quantity: 10
Welcome to Sorted Sally's Snack Bar.
0) Beans cost: $5.00 quantity: 1
1) Carrots cost: $2.00 quantity: 10
2) Slurm cost: $1.50 quantity: 3
Requirements/Hints:
-
Implement the function
insert_first
. This function should create a new snack struct and insert it at the beginning of the list. -
Implement the function
clear
. This function should clear and free all items in the list. -
Implement the function
printList
. This function should print the contents of the list. -
Implement three sort functions:
sortCost
andsortName
andsortQuantity
. These functions should sort by the given attribute.
4. Submit your Work
Before submitting, check that
-
Your programs run on goldengate using the
make
command! -
valgrind does not report any errors!
Push you work to github to submit your work.
$ cd A04 $ git status $ git add *.c $ git status $ git commit -m "A04 complete" $ git status $ git push $ git status
5. Grading Rubric
Assignment rubrics
Grades are out of 4 points.
-
(2 points) Magic Square
-
(0.1 points) style and header comment
-
(0.4 points) reads in matrix from stdin
-
(0.5 points) creates/deletes 2D matrix using malloc/free
-
(1.0 points) No memory errors
-
-
(2 points) Sorted Snacks
-
(0.1 points) style and header comment
-
(0.4 points) defines a struct correctly handles deletion and insertion to a linked list
-
(0.5 points) correctly sorts and displays snacks based on name, cost, or quantity
-
(1.0 points) handles file and linked list nodes correctly (no memory errors!)
-
Code rubrics
For full credit, your C programs must be feature-complete, robust (e.g. run without memory errors or crashing) and have good style.
-
Some credit lost for missing features or bugs, depending on severity of error
-
-5% for style errors. See the class coding style here.
-
-50% for memory errors
-
-100% for failure to checkin work to Github
-
-100% for failure to compile on linux using make