Assignment 9: Trust the Process
Due Friday, March 21, at midnight
The goals for this assignment are:
-
Gain experience working with
fork()
. -
Use OS system calls in your C program.
-
Implement a multi-process program
Update your repository
Do a fetch upstream to obtain the basecode for this assignment.
Using the command line
-
Open terminal and change your current directory to your assignment repository.
-
Run the command
git fetch upstream
-
Run the command
git merge upstream/main
Your repository should now contain a new folder named A09
.
The fetch
and merge
commands update your repository with any changes from the original.
1. Warm-up
In the file, warmup.c
, implement a program that spawns processes according to the following diagram.
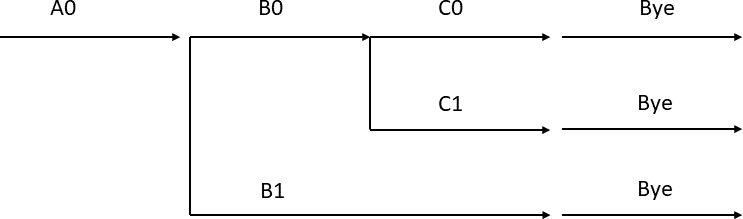
$ make warmup
gcc -g -Wall -Wvla -Werror warmup.c -o warmup
$ ./warmup
17300] A0
17300] B0
17301] B1
17301] Bye
17300] C0
17300] Bye
17302] C1
17302] Bye
Requirements:
-
Your program should print the messages as shown above.
-
Your program should use fork() to spawn processes.
-
Call
getpid()
to show the process ids for each print message.
2. Grep
In the file, grep.c
, implement a program that uses N processes to search for a keyword
in a set of files.
Your program implements a simplified version of the bash command grep
. Grep searches a list of files
for a given keyword or expression. For example, the following command searches for the keyword public
in a list of source files. We use the find
command to generate the list of source files to search.
For example:
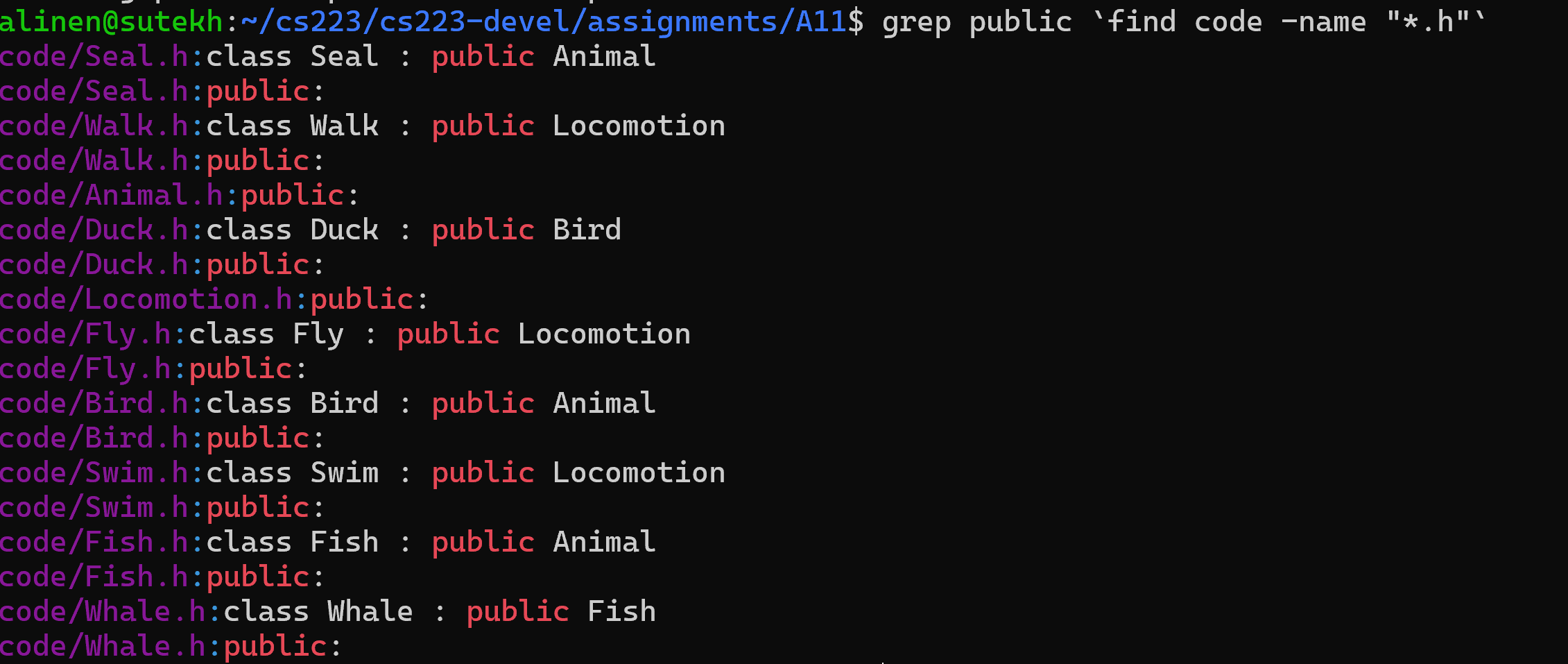
Make sure you include the backticks, e.g. ` . This adds the filenames found by find as command line arguments to grep .
|
$ make grep
gcc -g -Wall -Wvla -Werror grep.c -o grep
$ ./grep
usage: ./grep <
NumProcesses>
<
Keyword>
<
Files>
$ ./grep 1 class missing.txt
Searching 1 files for keyword: class
Process [613] searching 1 files (3 to 4)
Process [613] Error: Cannot open file
Total occurances: 0
Elapsed time is 0.000608
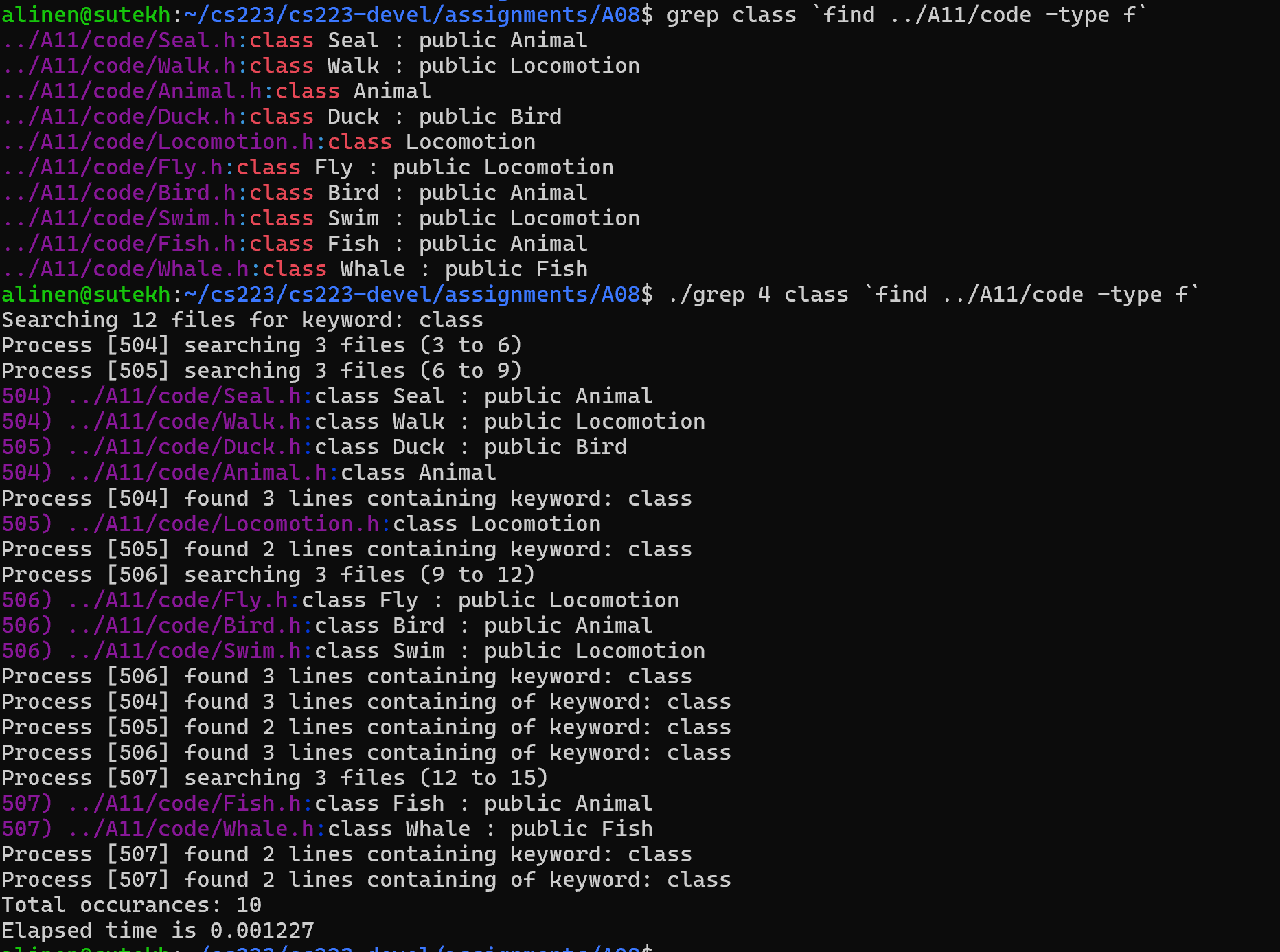
Requirements:
-
Your program should take the number of processes, keyword, and a list of files as command line arguments
-
Subdivide the files among the N processes. Note that the number of files may not divide evenly between processes.
-
Print the totals and sub-division of files similarly to the output above
-
Use the
fgets
function to process the lines in each file -
Use the function
strstr
defined instring.h
to search lines of text for the keyword. Documentation is here -
To compute the total count, each child process can return the number matching lines using its exitcode. Recall that the parent can use the status reported by
waitpid
to get the children’s exit code. -
Your program should report the amount of time needed to search using
gettimeofday
You can print with colors to the console using ANSI escape sequences (see below).
For example, printf(ANSI_COLOR_GREEN "Green Text" ANSI_COLOR_RESET); will print green text. Here is a good guide!
|
// Helpful macros for working with color #define ANSI_COLOR_RED "\x1b[31m" #define ANSI_COLOR_GREEN "\x1b[32m" #define ANSI_COLOR_YELLOW "\x1b[33m" #define ANSI_COLOR_BLUE "\x1b[34m" #define ANSI_COLOR_MAGENTA "\x1b[35m" #define ANSI_COLOR_CYAN "\x1b[36m" #define ANSI_COLOR_RESET "\x1b[0m"
3. Submit your work
Push your code to github to submit your work
$ git status
$ git add .
$ git status
$ git commit -m "assignment complete"
$ git status
$ git push
$ git status
4. Grading Rubric
Assignment rubrics
Grades are out of 4 points.
-
(1 points) Warmup
-
(0.1 points) style and header comment
-
(0.4 points) prints correct messages, uses
fork()
andgetpid()
-
(0.5 points) no memory errors
-
-
(3 points) Grep
Code rubrics
For full credit, your C programs must be feature-complete, robust (e.g. run without memory errors or crashing) and have good style.
-
Some credit lost for missing features or bugs, depending on severity of error
-
-5% for style errors. See the class coding style here.
-
-50% for memory errors
-
-100% for failure to checkin work to Github
-
-100% for failure to compile on linux using make