Assignment 10: Sending mixed signals
Due Friday, April 4th, before midnight
The goals for this assignment are:
-
Implement a program that uses signals to coordinate between two processes
-
Implement an embarrassingly parallel multi-threades algorithms using the pthread library
Update your repository
Do a fetch upstream to obtain the basecode for this assignment.
Using the command line
-
Open terminal and change your current directory to your assignment repository.
-
Run the command
git fetch upstream
-
Run the command
git merge upstream/main
Your repository should now contain a new folder named A10
.
The fetch
and merge
commands update your repository with any changes from the original.
1. Marco Polo
In the file, marco_polo.c
, implement a program that uses signals to coordinate between two processes, like so.
-
When the user presses the 'm' key, the parents sends SIGALRM.
-
When the child receives SIGALRM, the child prints "Marco" and sends SIGALRM to the parent.
-
When the parent receives SIGALRM, the parent prints "Polo".
You can use strace to track the signals sent between the the parent and the child.
$ strace -e 'trace=!all' -f ./marco_polo
Parent is 738
strace: Process 739 attached
m
[pid 739] --- SIGALRM {si_signo=SIGALRM, si_code=SI_USER, si_pid=738, si_uid=1000} ---
Marco [739]
[pid 738] --- SIGALRM {si_signo=SIGALRM, si_code=SI_USER, si_pid=739, si_uid=1000} ---
Polo [738]
m
[pid 739] --- SIGALRM {si_signo=SIGALRM, si_code=SI_USER, si_pid=738, si_uid=1000} ---
Marco [739]
[pid 738] --- SIGALRM {si_signo=SIGALRM, si_code=SI_USER, si_pid=739, si_uid=1000} ---
Polo [738]
q
[pid 739] --- SIGTERM {si_signo=SIGTERM, si_code=SI_USER, si_pid=738, si_uid=1000} ---
[pid 739] +++ killed by SIGTERM +++
--- SIGCHLD {si_signo=SIGCHLD, si_code=CLD_KILLED, si_pid=739, si_uid=1000, si_status=SIGTERM, si_utime=0, si_stime=0} ---
+++ exited with 0 +++
Requirements/Hints:
-
Use
fork
to create a parent and child process. -
Use
kill
to send signals between the parent and the child. -
Register two signal handlers, one for the parent and one for the child. Configure the signal handler to trigger when it receives SIGALRM.
-
The child process should say "Marco". The parent process should respond with "Polo".
-
In the parent, implement a loop that responds to commands from the user, specifically
-
'm' should send the initial signal that triggers the child to say "Marco"
-
'q' should send quit the application. This command should also send SIGTERM to the child, so the child also exits.
-
2. Grep
This question implements a simplified version of the bash command grep
. Grep searches a list of files
for a given keyword or expression. For example, the following command searches for the keyword public
in a list of source files. We use the find
command to generate the list of source files to search.
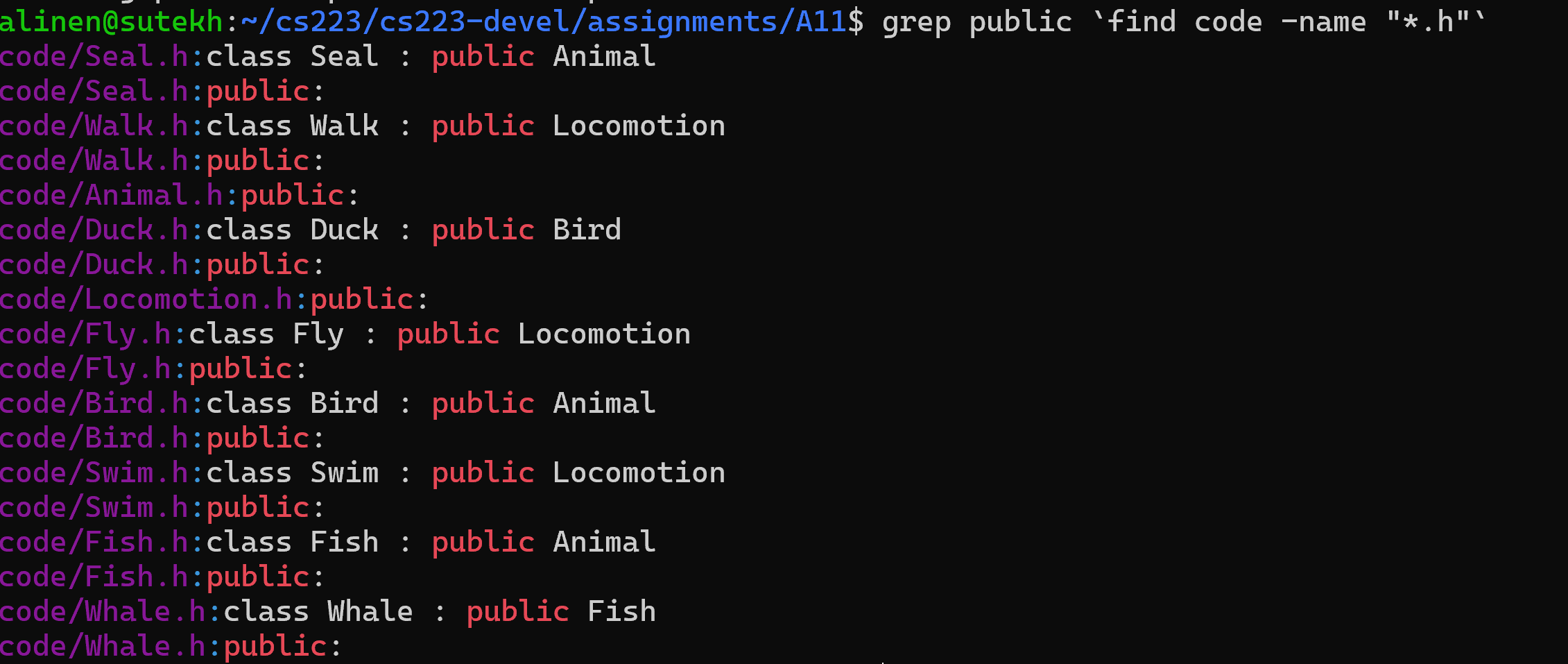
Make sure you include the backticks, e.g. ` . This adds the filenames found by find as command line arguments to grep .
|
In the file, grep.c
, implement a program that uses N threads to search for a keyword
in a set of files.
$ make grep
gcc -g -Wall -Wvla -Werror grep.c -o grep -lpthreads
$ ./grep
usage: ./grep <
NumThreads>
<
Keyword>
<
Files>
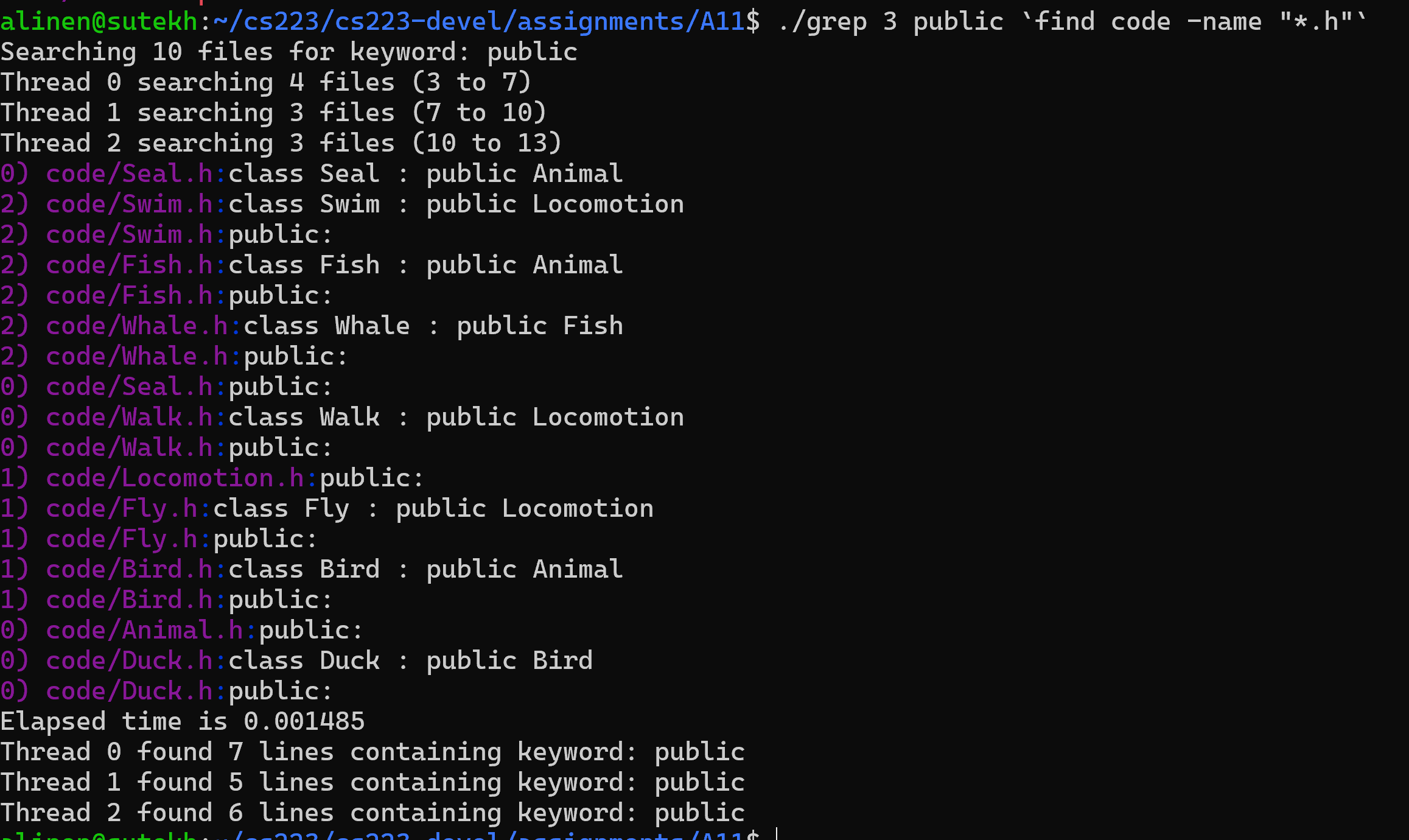
Requirements:
-
Your program should take the number of threads, keyword, and a list of files as command line arguments
-
Subdivide the files among the N threads. Note that the number of files may not divide evenly between threads.
-
Print the totals and sub-division of files similarly to the output above
-
Use the
fgets
to process the lines in each file -
Use the function
strstr
defined instring.h
to search lines of text for the keyword. Documentation is here
3. Submit your work
1) Push your code work to github
$ git status
$ git add .
$ git status
$ git commit -m "assignment complete"
$ git status
$ git push
$ git status
4. Grading Rubric
Assignment rubrics
Grades are out of 4 points.
-
(2 points) Marco Polo
-
(0.1 points) style and header comment
-
(0.9 points) Implementation satisfies requirements and works correctly.
-
(1.0 points) no memory errors
-
-
(2 points) Grep
-
(0.15 points) style and header comment
-
(0.1 points) Correct command line arguments: number of threads, keyword, and a list of files
-
(0.2 points) Subdivide the files among the N threads
-
(0.3 points) Correct output
-
(0.75 points) no memory errors
-
Code rubrics
For full credit, your C programs must be feature-complete, robust (e.g. run without memory errors or crashing) and have good style.
-
Some credit lost for missing features or bugs, depending on severity of error
-
-5% for style errors. See the class coding style here.
-
-50% for memory errors
-
-100% for failure to checkin work to Github
-
-100% for failure to compile on linux using make