Assignment 11: Glow
Due Friday, April 11th, before midnight
The goals for this assignment are:
-
Implement multi-thread algorithms using the pthread library
Update your repository
Do a fetch upstream to obtain the basecode for this assignment.
Using the command line
-
Open terminal and change your current directory to your assignment repository.
-
Run the command
git fetch upstream
-
Run the command
git merge upstream/main
Your repository should now contain a new folder named A11
.
The fetch
and merge
commands update your repository with any changes from the original.
Background
In this assignment, you will implement a glow filter for images. You will implement the filter both single- and multi-threaded.
Before:
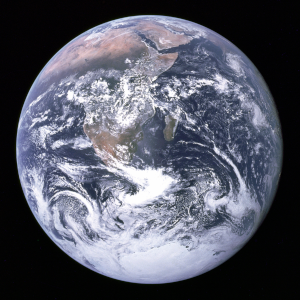
After:
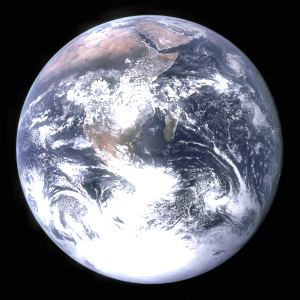
This filter requires three steps:
-
Extracting bright areas into a separate image
-
Blurring the bright pixels
-
Adding the blurred image to the original
We will work with PPM files for this assignment. |
Extract bright areas
For each pixel, you can compute its brightness as the average of its red, green, and blue values. E.g.
We can compare this brightness to a threshold, say 200, to determine if we should apply the glow to this area. For the image below, we iterated through every pixel in the original image, computed its brightness, and then saved its brightness to a new image if the pixel exceeded the threshold.
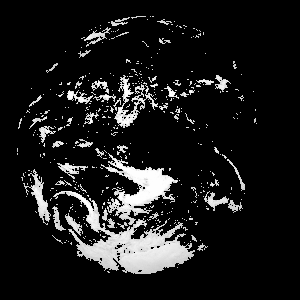
Blur
For this assignment, we will apply a box blur to each pixel in the image computed in the previous step.
In a box blur, the color of each pixel is set to the average of its neighbors. For example, if we look only at the immediate neighbors of the pixel (i,j), the new color would be as follows:
The above calculation would correspond to a box size of 3 because the region around the pixel has width 3 and height 3.
We compute the blur separately for each color channel (read, blue, green). In the image below, we used a box size of 25. In other words, we used the 24 neighbors that were left, right, above, and below of the current pixel. If a pixel is near the edge of the image, we can clamp the blur indices so they stay in the image.
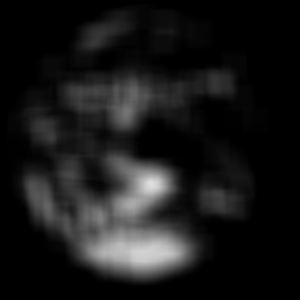
The glow will look better with a gaussian blur. Feel free to implement one instead |
Glow
The glow effect is achieved by adding the blurred image (bij) with the original image (oij).
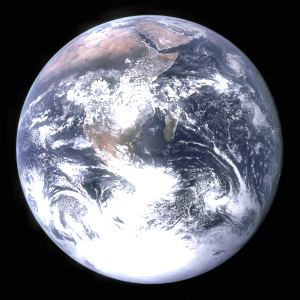
1. Single-threaded
In the file, glow.c
, implement a single-threaded program that applies a glow effect to the image "earth-small.ppm".
In this question, you will get the core algorithm working, before re-implementing it to be multithreaded in
the next question.
$ time ./glow
real 0m0.518s
user 0m0.513s
sys 0m0.001s
Requirements/Hints:
-
This program can be simple. You may hard-coded the input file (earth-small.ppm), the threshold (200), and the blur box size (25).
-
Your program must output a PPM file named
glow.ppm
-
Use software that can view PPM files to check your work, either via VS Code, eog (Eye of Gnome), Gimp, Photoshop, etc
2. Multi-threaded
In the file, glow_multithreaded.c
, implement a program that applies a glow effect using multiple threads.
It should run much faster than your single threaded version.
$ ./glow_multithreaded -h
usage: ./glow_multithreaded -N <NumThreads> -t <brightness threshold> -b <box blur size> -f <ppmfile>
$ time ./glow_multithreaded
Thread sub-image slice: rows (0,75)
Thread sub-image slice: rows (75,150)
Thread sub-image slice: rows (150,225)
Thread sub-image slice: rows (225,300)
real 0m0.249s
user 0m0.717s
sys 0m0.000s
Requirements and recommendations:
-
Each thread should run all steps on a portion of the image. I recommend you divide the image into horizontal slices (not square blocks)
-
You can assume that N will divide the images evenly.
earth.ppm
has resolution 3000x3000.earth-small.ppm
has resolution 300x300. -
Your program should output a file named
glow.ppm
-
Your program must support the command line arguments.
-
Your program may not use global variables.
3. Submit your work
Submit both your code and images.
1) Push your code work to github
$ git status
$ git add .
$ git status
$ git commit -m "assignment complete"
$ git status
$ git push
$ git status
4. Grading Rubric
Assignment rubrics
Grades are out of 4 points.
-
(4 points) Threaded Fractal
-
(0.4 points) style and header comment
-
(0.4 points) Generated image with correct filename
-
(0.2 points) Supports given command line arguments
-
(1.0 points) Correct output, thread coordination, and output performance statistics
-
(2.0 points) no memory errors
-
Code rubrics
For full credit, your C programs must be feature-complete, robust (e.g. run without memory errors or crashing) and have good style.
-
Some credit lost for missing features or bugs, depending on severity of error
-
-5% for style errors. See the class coding style here.
-
-50% for memory errors
-
-100% for failure to checkin work to Github
-
-100% for failure to compile on linux using make